728x90
반응형
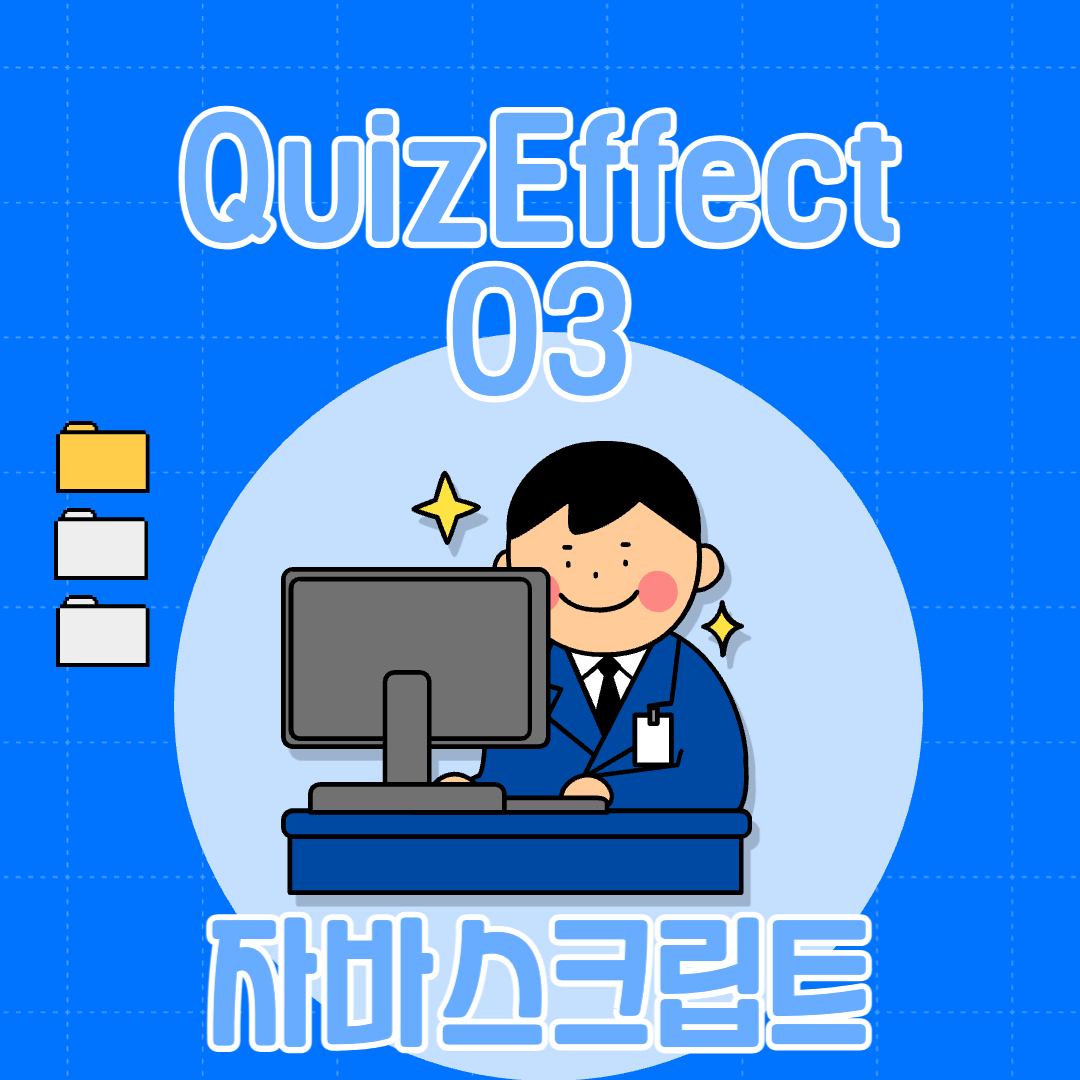
QuizEffect03
QuizEffect03 문제를 html, css, javascript로 만들어서 풀어봅니다.
선택자
querySelector를 사용하지만, 선택해야하는 것이 다중인 경우에 querySelectorAll을 사용합니다.
//선택자
const quizType = document.querySelectorAll(".quiz__type"); //퀴즈 종류
const quizNumber = document.querySelectorAll(".quiz__question .number"); //퀴즈 번호
const quizAsk = document.querySelectorAll(".quiz__question .ask"); //퀴즈 질문
const quizConfirm = document.querySelectorAll(".quiz__answer .confirm"); //정답 확인 버튼
const quizResult = document.querySelectorAll(".quiz__answer .result"); //정답 결과
const quizInput = document.querySelectorAll(".quiz__answer .input"); //사용자 정답
const quizView = document.querySelectorAll(".quiz__view"); //강아지
문제 정보
문제가 많아질 경우 변수를 선언해서 진행하면 더 효율적이다.
//문제 정보
const quizInfo = [
{
answerType: "웹디자인기능사 2015년 2회",
answerNum: "1",
answerAsk: "해질 무렵 정원을 바라보면 어두워짐에 따라 꽃의 빨간색은 거무스레해지고, 그것에 비해 나뭇잎의 녹색은 점차 뚜렷해짐을 볼 수 있다. 이것과 관련된 현상을 무엇이라고 하는가?",
answerResult: "푸르킨예 현상"
},
{
answerType: "웹디자인기능사 2015년 2회",
answerNum: "2",
answerAsk: "검색방식에서 두개 이상의 키워드 간의 관계를 설정하는 것은?",
answerResult: "연산자"
},
{
answerType: "웹디자인기능사 2015년 2회",
answerNum: "3",
answerAsk: "OSI 7계층 중 종단 간 오류제어와 흐름제어를 하는 것은?",
answerResult: "전송 계층"
},
{
answerType: "웹디자인기능사 2015년 2회",
answerNum: "4",
answerAsk: "IPv4에서 멀티캐스트를 위해 예약되어 있는 클래스는?",
answerResult: "클래스 D"
},
]
문제 출력
문제가 많아질 경우 for문, forEach문을 사용하면 진행하면 더 간단합니다.
//문제 출력
// quizType[0].textContent = quizInfo[0].answerType;
// quizType[1].textContent = quizInfo[1].answerType;
// quizType[2].textContent = quizInfo[2].answerType;
// quizType[3].textContent = quizInfo[3].answerType;
// quizNumber[0].textContent = quizInfo[0].answerNum + ". ";
// quizNumber[1].textContent = quizInfo[1].answerNum + ". ";
// quizNumber[2].textContent = quizInfo[2].answerNum + ". ";
// quizNumber[3].textContent = quizInfo[3].answerNum + ". ";
// quizAsk[0].textContent = quizInfo[0].answerAsk;
// quizAsk[1].textContent = quizInfo[1].answerAsk;
// quizAsk[2].textContent = quizInfo[2].answerAsk;
// quizAsk[3].textContent = quizInfo[3].answerAsk;
// quizResult[0].textContent = quizInfo[0].answerResul;
// quizResult[1].textContent = quizInfo[1].answerResul;
// quizResult[2].textContent = quizInfo[2].answerResul;
// quizResult[3].textContent = quizInfo[3].answerResul;
for ( i=0; i < quizInfo.length; i++ ){
quizType[i].textContent = quizInfo[i].answerType;
quizNumber[i].textContent = quizInfo[i].answerNum + ". ";
quizAsk[i].textContent = quizInfo[i].answerAsk;
quizResult[i].textContent = quizInfo[i].answerResult;
}
quizInfo.forEach((e, i) => {
quizType[i].textContent = quizInfo[i].answerType;
quizNumber[i].textContent = quizInfo[i].answerNum + ". ";
quizAsk[i].textContent = quizInfo[i].answerAsk;
quizResult[i].textContent = quizInfo[i].answerResult;
});
정답 숨기기
정답을 숨기는 것도 여러개의 경우 for문, forEach문을 이용하는 것이 효율적입니다.
//정답 숨기기(4개)
// quizResult[0].style.display = "none";
// quizResult[1].style.display = "none";
// quizResult[2].style.display = "none";
// quizResult[3].style.display = "none";
// for ( i=0; i < quizInfo.length; i++ ){
// quizResult[i].style.display = "none";
// }
quizInfo.forEach((e, i) => {
quizResult[i].style.display = "none";
});
// quizInfo.forEach((element) => {
// console.log(element)
// }); //작업하는 것을 확인하는 것이 콘솔로그. 첫번째 요소, 두번째 인텍스, 세번째 배열.
정답 확인
정답을 확인하기 위해 버튼을 클릭하면, 사용자의 정답과 입력한 값이 맞는지 확인하고 정답과 오답에 대한 정보를 표기합니다.
//정답 확인
// quizConfirm.addEventListener("click", () => {
// alert("ddd")
// }); //alert로 확인해 보는 것이 좋다. 정의되있지 않거나, 함수가 없을 때는 선택자를 의심해봐야함.
quizConfirm.forEach((btn, num) => {
btn.addEventListener("click", () => {
//사용자 정답
const userWord = quizInput[num].value.toLowerCase().trim(); //소문자로 바꾸고, 여백을 없애준다.
//사용자 정답 == 문제 정답
if(userWord == quizInfo[num].answerResult){
//정답
// alert("정답")
quizView[num].classList.add("like");
quizConfirm[num].style.display = "none";
} else {
//오답
// alert("오답")
quizView[num].classList.add("dislike");
quizConfirm[num].style.display = "none";
quizResult[num].style.display = "block";
quizInput[num].style.display = "none";
}
})
});
728x90
반응형
'Effect' 카테고리의 다른 글
searchEffect02 (2) | 2022.08.17 |
---|---|
searchEffect01 (4) | 2022.08.17 |
QuizEffect04 - 객관식(한문제) 유형 (9) | 2022.08.08 |
QuizEffect02 - 주관식 유형 (4) | 2022.08.04 |
QuizEffect01 - 정답 확인 유형 (4) | 2022.08.04 |